What is Typescript?
Typescript is a superset of Javasript, created with the goal of making the language more reliable and scalable. It is developed using the same syntax and semantics as Javascript, enabling developers to use existing Javascript code. A language is a superset of another if it contains all of the features of a given language and has been expanded or enhanced to include other features as well. It can be called from from Javascript and incorporates popuplar Javascript libraries. This also means that Javascript is Typescript. All *.js files can be renamed *.ts and compiled [5].
This language is typed, enabling developers to statically verify code. A type, also known as a data type, identifies and classifies various types of data (e.g. Integers, String, Floats, and Booleans). Type checking is the process of verifying the limitations related to various types. In Typescript’s case, type checking occurs at compile time, and is therefore static. This is in opposition to dynamic type verification which occurs at runtime. An advantage of type checking is that the compiled code executes much more quickly, as the compiler knows the exact data types that are in use. [3, 5]
The typescript compiler is a source-to-source code compiler, known as a transpiler. It creates a copy of the *.ts file in javascript.
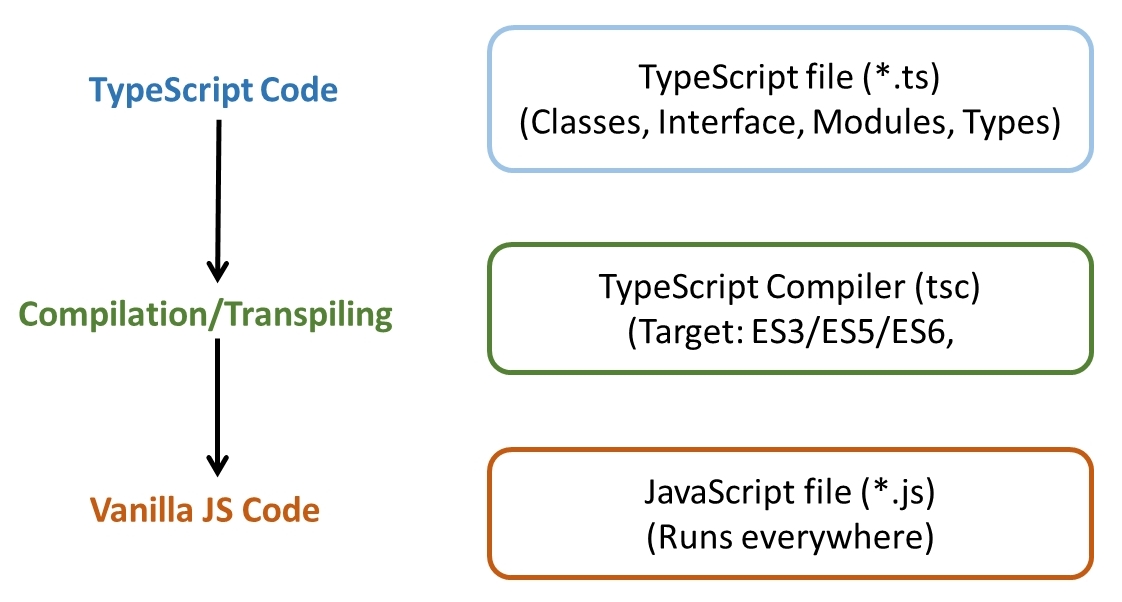
It is an open-source language that has been maintained by Microsoft since it’s creation in 2012. It’s widespread use as a core programming language is said to have occured due to it’s utilisation as a core programming language in Angular 2.
This language compiles to Javascript, enabling it to run on any browser. [5]